Exception handling in PHP
What is an exception?
An exception indicates that there is a problem while running an application.
Here’s a quote from Wikipedia on exception handling:
Exception handling is a programming technique that allows a programmer to control errors that occur during the execution of a computer program.
(wikipedia.org)
So, how do we handle exceptions in PHP?
In PHP, we can throw an exception using the throw
keyword and catch it with catch
.
To make it easier to handle exceptions, it is recommended to place the code where the error might occur inside a try
block, which must have at least one catch
or finally
block, or both.
Let’s go over each of these blocks:
- try: Contains the code that may cause a possible exception. This code is executed unless an exception is thrown.
- throw: With
throw
, we can trigger an exception. This means that even if the code doesn’t technically have an exception, we define it as such. - catch: The
catch
block catches the exception. Insidetry
, we can have multiplecatch
blocks, allowing us to capture different exceptions and handle each accordingly. - finally: This block is executed whether or not an exception is found. Similar to
catch
, there can be more than onefinally
block.
Example with throw
: We’ll create an exception no matter what.
<?php try{ throw new Exception('una excepcion sin sentido'); }catch(Exception $e){ echo "la excepcion capturada es: ".$e; } ?>
Alright, let’s see what we get on the screen.

Example without throw
: let’s divide by 0.
<?php try{ $x =1/0; echo $x; }catch(Exception $e){ echo $e; }finally{ echo "<br/> no debes dividir por 0"; } ?>
In this case, we get the following exception:
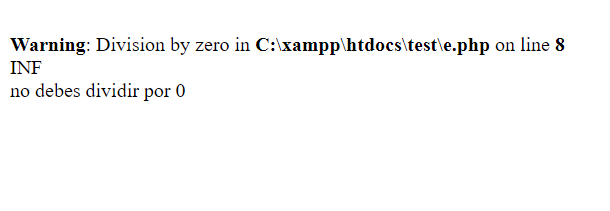
Reference: php.net
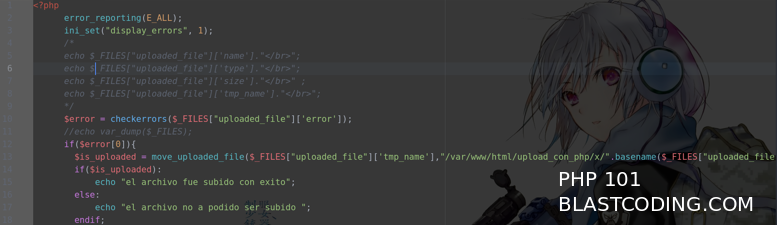
- Anterior: Errores en PHP
- Siguiente: Generadores en PHP