PHP routing
The routing in PHP is the practice of defining how URLs will be associated with actions we perform in our web application. This is useful when using the MVC design pattern, for example: I enter the main page /, call the controller that will determine what to display, a landing page, an introduction, or perhaps a list of the latest topics.
You probably won’t find much about this topic on the official PHP page or other places that discuss PHP, but you will find it in frameworks. I think the first time I came across this was in a book on Modern PHP or something similar.
Do you remember this MVC diagram?
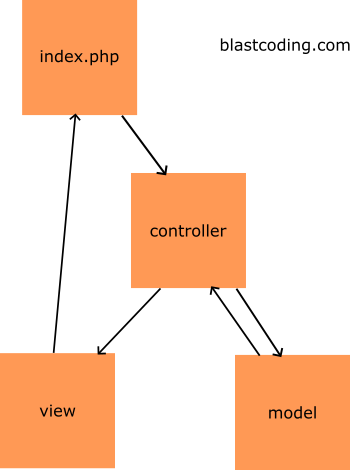
Maybe you’re wondering, “How does everything we write in our browser go through index.php when we use an MVC framework?”
Redirection to index.php
This happens when we modify the .htaccess file or define the behavior to redirect on our server so that it redirects to index.php.
.htaccessRewriteEngine On RewriteBase / RewriteCond %{REQUEST_FILENAME} !-f RewriteCond %{REQUEST_FILENAME} !-d RewriteRule ^.*$ /index.php [L,QSA]
These rewriting rules are commonly used to redirect all requests that do not correspond to existing files or directories to a file index.php, which is typically the entry point of the web application. This is typical in controller-based routing in PHP frameworks.
But before that, to simulate a real server, we should create a virtual host. If you want to do this, you can find more information at https://blastcoding.com/configuracion-de-virtual-host-en-apache-windows/. In that post, Apache from XAMPP is used to create a virtual host.
If we don’t create this virtual host, when we bring everything to the index, it will show the localhost dashboard.
Routes
Once we have our host, we can create our index.php. But what does our index.php need for routing?
Well, the first thing the index needs is routes: the simplest way to create this is with an array, although there are other ways to create routes.
What should our routes contain?
First and foremost, it should have the path we’ll enter in the browser, and secondly, where this route will lead. For example:
$routes = [ "/" => "PageController/index", "patita" =>"PageController/patita", "/user/register" => "UserController/register" ];
Routing
And how do I know that a route is being used? How do I get the route I’ve written in the browser?
For this, we’ll have to use $_SERVER["REQUEST_URI"]
. If I do the following:
//supongamos que mi virtualhost es miproyecto.local //si le pasamos miproyecto.local/pepe/www echo "<p>".$_SERVER["REQUEST_URI"]."<p>";
/pepe/www// mostrara en pantalla
This is the URI that I just passed; now I should be able to process it. For that, we could check if the route exists beforehand using array_key_exists
:
$route_key = array_key_exists($URI,$routes); if($route_key){ echo "existe"; //codigo para procesar la ruta }else{ echo "No exite: mandar a una pagina 404 o cargar una view que presente pagina no encontrada"; //header('Location: 404.php'); }
Let’s try to do simple routing without the MVC design pattern; let’s change the routes a bit:
Ejemplo$routes = [ "/" => "PageController/index", "/patita" =>"PageController/index", "/user/register" => "UserController/register", "/dashboard" =>"dashboard.php" ]; $URI = make_URI_valid($_SERVER["REQUEST_URI"]); $route_key = array_key_exists($URI,$routes); if($route_key){ header("Location: ".$routes[$URI].""); }else{ header('Location: 404.php'); }
We create 2 simple files, dashboard.php and 404.php, and our routes should already work. The function make_URI_valid
is a function I created to make the routes consistent so that when there is no route, it adds /.
Exercises
Exercise 1:
Use a routing package from a third-party, such as Symfony or another. You can also create an MVC structure based on this. You will need to know about namespaces and Composer to complete this exercise.
Exercise 2:
Create your own routing based on this and build a website that uses a simple MVC design pattern, or at least the controller part. This exercise can be challenging, but it will make your brain work.
You will need to know about namespaces and Composer to complete this exercise.
Here’s another way to do routing: https://gist.github.com/shinigamicorei7/8cccbcfc9eb6f234d8d8. In this case, they are doing routing with a class. Note that in this post, we want you to understand how routing works. You will generally use routes provided by a framework.