PHP making directories with mkdir function
To create a directory in PHP, you use the mkdir()
function, which can accept up to four parameters. The basic syntax is as follows:
mkdir ( string $pathname [, int $mode = 0777 [, bool $recursive = FALSE [, resource $context ]]] ) : bool
The content you provided explains the mkdir()
function in PHP well. Here’s a quick summary to ensure clarity:
- $pathname: Specifies the full path to the directory you want to create.
- $mode: Represents the permission settings for the directory, defaulting to
0777
. However, this value can be affected by the server’sumask
. - $recursive: If
true
, this allows the creation of nested directories. Iffalse
, only the specified directory will be created. - $context: (Optional) This is a resource that allows the behavior of
mkdir()
to be altered. Typically not needed for basic use cases.
Additionally, it’s important to ensure that your web server (e.g., Apache or Nginx) has the correct write permissions to the directory in question. If not, you may face a “Permission denied” error, as you mentioned.
Your explanation is thorough, and highlighting the importance of server permissions is a good touch. It might also help to mention that users can troubleshoot this error by adjusting file system permissions or ownership using chmod
or chown
.
PHP mkdir: Permission denied problem.(Linux), on Windows will work correctly.
In the case of Linux, we would need to do the following:
Make sure all files are owned by the Apache group and user. In Ubuntu, it is the www-data
group and user:
chown -R www-data:www-data /path/to/webserver/www
Next, enable all members of the www-data
group to read and write files:
chmod -R g+rw /path/to/webserver/www
Let’s do an example without permissions:
First, I will create a folder for this example just like I would on my LAMP (Linux Apache PHP) server. So I will go to my folder /var/www/html/
and create a folder called create_file_con_php
.
mkdir create_file_con_php
Inside the folder create_file_con_php, I will create two PHP files just to show that the folder will be created when I run the file file.php.
In the file index.php, I will create a form to allow users to create the folder they want.
/var/www/html/create_file_con_php/index.php<html> <head> <title>Crear un directorio o carpeta con PHP</title> </head> <body> <h1>Creacón de directorio o carpeta con php</h1> <form action="file.php" method="post"> <input type="text" name="name" placeholder="nombre de directorio"/> <input type="submit" value="submit"> </form> </body> </html>
Finally, in file.php I will use mkdir
to create the directory
<?php error_reporting(E_ALL); ini_set("display_errors", 1); $result = mkdir($_SERVER['DOCUMENT_ROOT']."/create_file_con_php/".$_POST['name']); if(!$reult): echo "no pudo crear la carpeta</br>"; echo $_SERVER['DOCUMENT_ROOT']."/create_file_con_php/".$_POST['name']."</br>"; else: header("Location: index.php"); endif; ?>

Now we’ll apply what was previously explained about permissions on Linux. In my console, I will enter the following lines and execute them.
/var/www/html/sudo chown -R www-data:www-data /var/www/html/create_directory_con_php sudo chmod -R g+rw /var/www/html/create_directory_con_php
On this moment or little script would work, we check, and it’s done we have a folder now, we have our directory created.
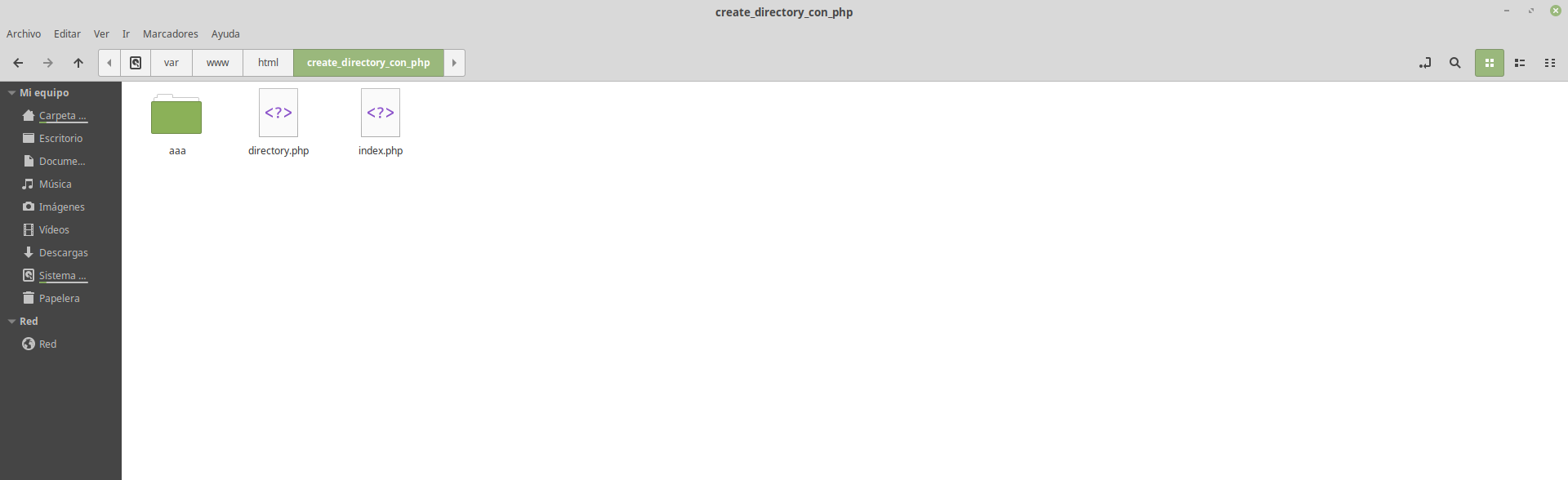
Using file_exists
If we want to create something more elaborate, we can use the file_exists
function.
file_exists(string $filename) :bool
This function receives a string, which is the path or route to the folder. In my case, it would be: "var/www/html/create_directory_con_php/nombre_de_la_carpeta"
Obviously, this function returns a boolean, indicating whether it exists or not. Let’s see how the directory file turned out.
directory.php<?php error_reporting(E_ALL); ini_set("display_errors", 1); $path = $_SERVER['DOCUMENT_ROOT']."/create_directory_con_php/".$_POST['name']; if(file_exists($path)){ echo "la carpeta a crear ya existe<br/>"; echo "<a href=\"index.php\"> <- back</a>"; }else{ if(create_directory($path)){ header("Location: index.php"); }else{ echo "no pudo crear la carpeta</br>"; echo $path."</br>"; } } function create_directory($path){ $result = mkdir($path); if(!$result): return false; else: return true; endif; } ?>Descargar repo en github
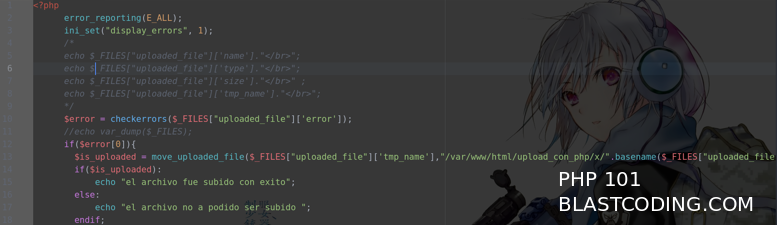
- Anterior: Interfaces y clases predefinidas
- Siguiente: Subiendo archivos a nuestro servidor