PHP Clean Code
There are quite a large number of tools for creating clean PHP code. In fact, people often complain that the code is not clean due to the code embedded in HTML.
The code embedded in HTML is seen a lot in PHP, yes. The code can be made much clearer by separating both things as well. PHP allows for these things and people tend to abuse it.
PHP offers its programmers a large number of tools that allow them to write really clean code if they so desire. Among these tools we have:
- functions
- classes
- traits
- Heredoc y Nowdoc
- include, include_once, require, requrie_once and partial parts(components)
- routing
- composer
- third party templates like blade.
- MVC architectural design pattern
- Frameworks
- others
So we have a lot of things we can do to improve.
Heredoc
It’s a shame that heredoc is never used. I’ve never seen anyone using it. In fact, the first time I used heredoc was when creating the template for this blog.
With PHP we can do something like the following:
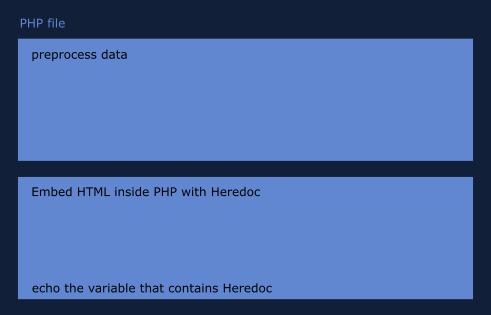
And at the end of all this, we do an echo
to display it on the screen. Let’s see a more elaborate example:
In the following example, I have used Bootstrap for the page in a faster way and with some style.
This will be our main.php so that you have an idea of the power we have with heredoc:
main.php//blastcoding.com <?php //preprocess variables and code $title = "This is my main text"; $main_text ="Phasellus non ornare justo. Duis vulputate ipsum ac malesuada ornare. Nunc faucibus quam vitae gravida efficitur. Quisque quis justo in nunc maximus ultricies. Quisque nec sagittis turpis. Sed ultricies sed lacus et aliquet. Sed dictum pretium neque et facilisis. Praesent eget diam eu odio imperdiet consectetur vitae a leo. In ullamcorper dolor vel fermentum convallis. Donec rhoncus tempus finibus. Aenean lectus lorem, interdum vitae accumsan sit amet, facilisis at augue. Donec suscipit feugiat mi a fermentum. Nam quis felis sit amet est euismod lacinia eu a enim. Sed sed ligula urna."; //template $main =<<<HTML <div class="container"> <div class="row"> <div class="col-8"> <main> <h1>$title</h1> <p> $main_text </p> </main> </div> <div class="col-4"> </div> </div> </div> HTML; //render echo $main;
You can see more about heredoc in:
Invoked immediately functions
The functions that run immediately can be of great help in PHP. I realized this when creating the WordPress theme currently in use on the blog. Let’s recall the previous theme that used Heredoc; it has a small issue in that we are using variables that might overwrite others.
This is where the invoked immediately functions come in. Suppose we come from another page that passes data to our view or template as an array, as we may want to call it. We can make a function that runs immediately receive this data as a parameter.
Within the function, we can create variables that undergo pre-processing before using them in the template.
For example:
Invoked immediately function reciviendo datos$se= "lateral goes here"; (function() use($se){ //preprocess variables and code $title = "This is my main text"; $main_text ="Phasellus non ornare justo. Duis vulputate ipsum ac malesuada ornare. Nunc faucibus quam vitae gravida efficitur. Quisque quis justo in nunc maximus ultricies. Quisque nec sagittis turpis. Sed ultricies sed lacus et aliquet. Sed dictum pretium neque et facilisis. Praesent eget diam eu odio imperdiet consectetur vitae a leo. In ullamcorper dolor vel fermentum convallis. Donec rhoncus tempus finibus. Aenean lectus lorem, interdum vitae accumsan sit amet, facilisis at augue. Donec suscipit feugiat mi a fermentum. Nam quis felis sit amet est euismod lacinia eu a enim. Sed sed ligula urna."; //template $main =<<<HTML <div class="container"> <div class="row"> <div class="col-8"> <main> <h1>$title</h1> <p> $main_text </p> </main> </div> <div class="col-4"> $se </div> </div> </div> HTML; //render echo $main; })();
For example, you can use this in CodeIgniter. This is faster than using a template and also faster than constantly opening and closing PHP tags like crazy
Components(Partial parts)
PHP provides us with a number of tools for including code within another, these are include
, include_once
, require
, require_once
.
It’s a good idea to separate the header, footer, and other parts of the page so that they are easier to address.
This way we will have a folder called partials, components, or parts which will have fragments of the page. Additionally, having the sections separated makes it easier to change the different visual parts of our project.
There is no point in having a part that repeats constantly, just create it and include it as part of the view.
For example, suppose that our index.php could be as follows:
index.php<!doctype html> <html lang="EN"> <head> <?php require_once("partials/head.php"); ?> </head> <body> <?php require_once("partials/header.php"); require_once("partials/main.php"); require_once("partials/footer.php"); require_once("partials/scripts.php"); ?> </body> </html>
If we look closely, we have the code fragments that will be part of the index in the partials folder. This way, for example, if we are asked to have a different header, we can go and modify the header.php.
In a large project, this would be a view and not an index.php. In a large project, we would use a design pattern called MVC, which is what we will see next quickly.
MVC
Another way to make our PHP code cleaner is to use the MVC design pattern.
The MVC design pattern, explained in simple terms, is a way of dividing our code into 3 parts: the view, which is what we will show to the user, the model that will communicate with our database, and the controller that will be responsible for calling the view and doing pre-processes before sending information to the model.
If you want to see it in a more formal way, here is the definition given to us by Wikipedia:
Model-view-controller (MVC) is a software architectural pattern, which separates data and mainly the business logic of an application from its representation and the module in charge of managing events and communications. To do this, MVC proposes the construction of three distinct components: the model, the view, and the controller; that is, it defines components for the representation of information and, on the other hand, for user interaction. wikipedia.com
Usually, our project will be divided into 3 main folders, an index.php, and we will use Composer to use autoload to simplify things.
Also within MVC, we could have routing within the index.php file.
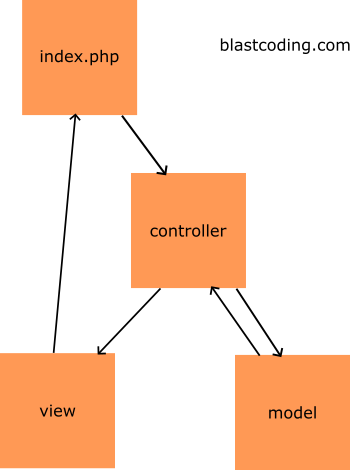
The structure of a modern MVC will generally be as shown in the previous image. Routing from index.php is used in many frameworks such as Laravel, CodeIgniter, and others, and even in CMS like WordPress.
Usually, the main things to start building an MVC project from scratch will be:
- src(non public folder)
- models
- users.php(class)
- products.php(class)
- views
- components(partials)
- user.php
- register.php
- login.php
- products.php
- controllers
- UserController.php(class)
- ProductController.php(class)
- models
- public(the public folder)
- vendor
- composer.json
- index.php(routing)
In general, our project would be structured something similar to what was previously explained. You can take a look of how CodeIgniter does this.
Frameworks
Currently, we would use a PHP framework to simplify the process of creating an MVC from scratch. Some of the frameworks you could use are Laravel, Symfony, CodeIgniter, CakePHP, and others.
Laravel is the most popular framework today, closely followed by CodeIgniter. Symfony is a framework that you can use for large websites.
Yii2 is another good framework that you could use.
Conclusión de Código limpio en PHP
In conclusion, we can see that there are many ways to make our code cleaner in PHP.
- The MVC design pattern offers us an important way to separate the logical part from the visual part. In this post, we did not see how to create controllers and models because it will depend on the framework you use or if you create it from scratch.
- Heredoc is a very useful tool for separating our PHP from HTML code.
- Separating our views into different components makes the code easier to manage, so we can modify parts of the views without them being a headache.
- The use of Composer and its autoload is almost mandatory today. In this way, we can better use MVC.
- Laravel is the most popular framework today.
As you can see in our heredoc, we use the term <<<HTML at the beginning and HTML; at the end, although the name we give it shouldn’t make a big difference, it does in the IDE. For example, I use Visual Studio Code. When we use the close tag and end tag with the name HTML, this IDE treats it as if it were HTML, and therefore colors all HTML syntax except PHP variables.