Handling URL Parameters with $_GET in PHP
In this article, we will look at how $_GET
works in PHP. $_GET
is an associative array of variables passed to the current script via URL parameters.
Let’s see a really simple example. Below, we’ll create a form that passes a name via URL to the same path using $_SERVER['PHP_SELF']
.
In my case, I have created all the examples in a folder tutorialphp.
$_GET<?php $form =<<<HTML <form method="GET" action="{$_SERVER['PHP_SELF']}"> <input type="text" name="name" placeholder="write your name here"> <input type="submit" value="confirm"> </form> <hr/> HTML; echo "<h1>Ejemplo de $_GET -Blastcoding.com</h1>"; echo $form; if(isset($_GET['name'])){ echo "hola {$_GET['name']}"; }
Notice that in the URL, for example, if I entered ‘Luis’ in the form, the following URL will appear after running it:
http://localhost/tutorialphp/get.php?name=Luis
Okay, but what happens if we have more than one variable?
Let’s go back to the form and add one more input, in this case, the last name.
$form =<<<HTML <form method="GET" action="{$_SERVER['PHP_SELF']}"> <input type="text" name="name" placeholder="write your name here"> <input type="text" name="surname" placeholder="write your surname here"> <input type="submit" value="confirm"> </form> <hr/> HTML; echo "<h1>Ejemplo de $_GET -Blastcoding.com</h1>"; echo $form; if(isset($_GET['name']) && isset($_GET['surname'])){ echo "hola {$_GET['name']} {$_GET['surname']}"; }
For example, in this case, if I write ‘Alan’ in the name field and ‘Wake’ in the surname field, how will our URL look?
Our URL will be as follows:http://localhost/tutorialphp/get.php?name=Alan&surname=Wake
As we can see, our variables in the URL are separated from the path to the page by ?
and between each other by &
.
Now, enter the following URL in your browser, replacing the name and surname with whatever you like. In my case, I will use ‘Wolo’ for the name and ‘lo’ for the surname:
http://localhost/tutorialphp/get.php?name=Wolo&surname=lo
The result will be that ‘Wolo lo’ is written on the screen of our browser because in our PHP file, we will receive the values of name
and surname
in the superglobal $_GET
.
As you might be thinking, this method of passing data allows users to pass values through the URL.
Uses
Now, this is all well and good, but you should keep in mind that the data the user is allowed to input should not be personal information, such as passwords, usernames, or sensitive details.
Remember that these data remain in the browser history, and we should also check that these inputs aren’t open for anyone to manipulate.
On the other hand, a useful place to apply this is in the search functionality of our program. In fact, there’s a CMS that uses this: WordPress. There’s an article on WordPress search that might interest you.
Security
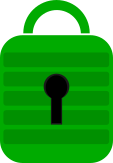
On the other hand, we must keep in mind that any place where we allow users to input information can be an entry point for someone with malicious intent trying to hack our site.
This might not be immediately obvious. You may ask, ‘How can someone hack my site through a search field?’ Well, it’s possible. Keep in mind that if we’re making an SQL query, which could allow an attacker to use SQL injection on our site, potentially gaining access to sensitive information or, in the worst case, deleting our database.
To prevent SQL injection or XSS attacks, it’s best to validate the data we receive as input, use stored procedures, views in SQL, or similar practices in the language or framework we’re using.
We can avoid this by correctly using the prepare
and query
methods within PHP’s PDO extension or the MySQLi extension.
Additionally, as we previously mentioned, we could use SQL stored procedures and views, but it’s generally more recommended to use the prepare
and query
methods.
To prevent XSS attacks, we can use CSRF protection in PHP and also implement CSP (Content Security Policy). Keep in mind that CSP is not foolproof, so using CSRF is also recommended.
These two forms of protection can be seen as layers of security; one doesn’t replace the other.