PHP data types
In this section, we will explore all the data types in PHP. Understanding these data types is fundamental to working effectively with PHP, as each type serves a different purpose and has unique characteristics. From basic types like integers and strings to more complex structures like arrays and objects, we’ll cover them all and provide examples to illustrate how they work in practice
Below is a list of the possible types of variables in PHP:
- Booleans
- Integers
- Float
- String
- Arrays
- Iterables
- Objects
- Resources
- Null
Related
- Callbacks
- Pseudo-types
- Type Juggling
- Cast
- Enumerations
booleanos
A boolean variable type can hold either a true or false value.
<?php $bool1 = true; //$bool1 is a variable width data type boolean with true as value $bool2 = false;//$bool2 is a variable width data type boolean with false as value ?>
Integers
An integer is a number that belongs to the set ℤ = {…, -2, -1, 0, 1, 2, …}.
Examples of declarations:
<?php $entero = 42; echo $entero; $entero = 01; echo $entero; $entero = 0x22; echo $entero; $entero = 0b11;// si mal no me salen las cuentas esto es 3 ?>
Floats( float / double )
Floating-point numbers can be any type of number; they belong to the set of real numbers.
Example: 2.3, 3.33333.
String
These are all variables of type text, a series of characters up to 256 bytes. Since PHP 7.0, the series of characters can exceed 256 bytes, and there doesn’t seem to be any restrictive limitation.
<?php echo 'echo'; echo "ejemplo con comillas dobles"; ?>
Let’s look at an example to better understand the difference between double quotes and single quotes.
diferencia entre comillas dobles y simples$value = 'something from somewhere'; echo "mi valor es $value \n"; echo 'mi valor es $value \n'; echo ": no hay salto de linea";
mi valor es something from somewhere mi valor es $value \n: no hay salto de linea
Notice that within single quotes, no parsing occurs, while within double quotes, the value of $value will be taken where this variable is found.
Array
I’m not sure if I can really classify an array as a variable type, since it is actually an ordered map. Ordered maps associate variables with keywords (keys). For example, associating ‘a’ with the value ‘b.’
An array can contain another array, trees, and even multidimensional arrays. <Ver más>
<?php $a = array( "foo" => "bar", "bar" => "foo" ); ?> echo $a['foo'];// devolvera bar echo $a['bar'];//devolvera foo
Iterables
Since PHP 7.1, there is an iterable variable type. This accepts either an array
or an object
that implements the Traversable interface. This interface detects if a class can be iterated over using foreach
.
It can be used with yield from within a generator.
use:
It can be used as a parameter in a function, and if it is not iterable, it will throw a TypeError
.
<?php function foo(iterable $iterable) { foreach ($iterable as $value) { // ... } } ?>
Objects
Let’s better understand what an object is through the definition from Wikipedia.
An object in OOP (Object-Oriented Programming) represents a real-life entity, meaning one of the objects related to the business we are working with or the problem we are facing, and with which we can interact.
By studying them, we gain the necessary knowledge to group them according to their characteristics through abstraction and generalization. These groups determine the classes of objects we are working with.
Let’s create a house object, just like in the initial example.
Functions within the object are called methods
<?php class Casa { private $ventanas = 5; private $puertas = 3; function mostrar() { echo "tengo una casa con $this->ventanas ventanas y $this->puertas puertas"; } public function setVentanas($nro){ $this->vetanas = $nro; } public function setPuertas($nro){ $this->puertas = $nro; } } $miCasa= new Casa; $miCasa->mostrar(); // puede usar las funciones setVentana y setPuertas para setear la cantidad de ventanas y puertas ?>
I will talk more about classes in the section on objects and classes in PHP
Resources
Resource variables contain references to external resources, and they are created and used by special functions.
Examples: A MySQL link is used to connect to a database. Generally, you won’t interact with these variables directly, but through a function. In this case, mysql_connect()
creates the resource, which can be used with other functions (e.g., mysql_query()
, mysql_result()
, and others) and is destroyed by mysql_close()
.
NULL
The special value Null represents a variable with no value, and this is the only value that this type of variable can have.
CALLBACKS – Callable functions
Some functions in PHP accept functions as parameters. These functions can be user-defined and are known as callbacks.
Let’s take an example of a PHP function that accepts another function as a parameter.
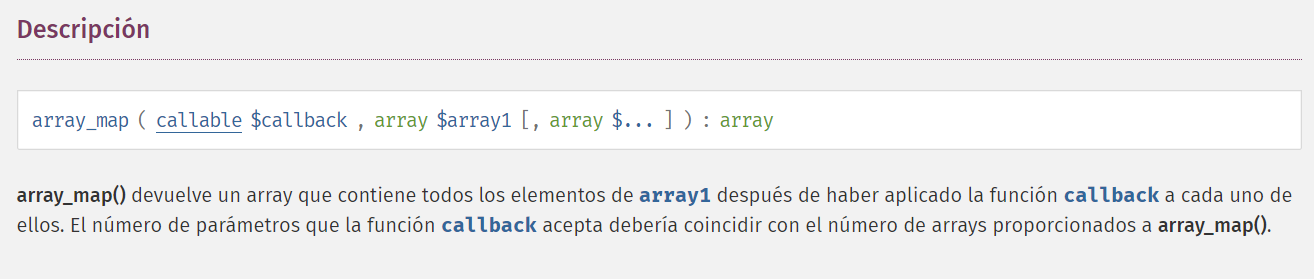
<?php $myfunction = function($a):int{ return $a+2; }; $b = array_map($myfunction, [1,2,3,4]); foreach($b as $c){ echo "$c<br/>"; } ?>
Well, I went a bit overboard with the example. What it’s doing is adding +2 to the values in array_map
through our callback function. Then, it prints it on the screen by iterating over the array $b
with foreach
.
Pseudo – types
Pseudotypes are keywords used in PHP documentation to specify the types or values that an argument can have. Please note that they are not primitives of the PHP language. Therefore, you cannot use pseudotypes as type hints in custom functions.
This has changed recently; see functions in PHP.
Type Juggling
PHP does not require (nor support) the explicit definition of types in variable declarations.
The type of the variable is determined by the context in which the variable is used. That is, if a string value is assigned to a variable $var
, then $var
becomes a string.
If an integer value is then assigned to the same variable $var
, it becomes an integer.
<?php $foo = "0"; // $foo es string (ASCII 48) $foo += 2; // $foo es ahora un integer (2) $foo = $foo + 1.3; // $foo es ahora un float (3.3) $foo = 5 + "10 Cerditos pequeñitos"; // $foo es integer (15) $foo = 5 + "10 Cerdos pequeños"; // $foo es integer (15) ?>
PHP Data types conversion(cast)
We can convert to a specific type or force it to be a specific type using parentheses ()
(int)
, (integer)
– cast to integer
(bool)
, (boolean)
– cast to boolean
(float)
, (double)
, (real)
– cast to float, double or real
(string)
– cast to string
(array)
– cast to array
(object)
– cast to object
(unset)
– cast to NULL
Enumerations
a partir de PHP 8.1It serves as a restrictive layer over classes or class constants, providing a way to define a set of possible values for a variable type. Briefly, we are creating a variable type that can accept certain values.
<?php enum Suit { case Hearts; case Diamonds; case Clubs; case Spades; } function do_stuff(Suit $s) { // ... } do_stuff(Suit::Spades); ?>
In fact, we have been using an enumeration class since the early days of PHP, and this is boolean
, which is a built-in enumeration (that comes by default in the language or program) that allows us 2 values: true
or false
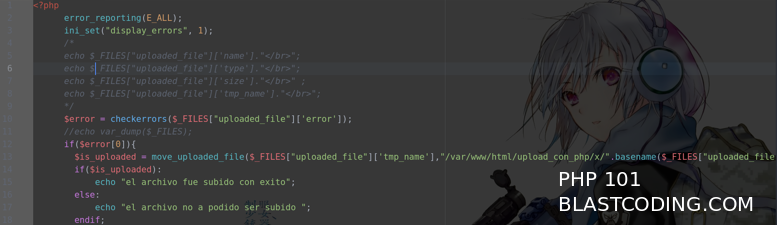
- Anterior: Sintaxis en PHP
- Siguiente: Variables en PHP