Creating files in PHP
We will see how to create files in PHP in different ways. Below is a list of the content in this entry.
Content:
- Introduction
- Creating a file with
fopen()
- Reading a file with
fread()
- Writing to a file with
fwrite()
- Creating a file with
file_put_contents
Intro
https://blastcoding.com/en/creating-files-in-php/#introIn PHP, there are two ways to create files. The first is by opening and closing the file manually, like in many other programming languages, and the second is by using a different PHP function that handles it automatically.
Let’s start with the first method: using fopen().
Creating the file with fopen()
https://blastcoding.com/en/creating-files-in-php/#fopenAlthough in this post we will use the fopen
function to create the file, keep in mind that this function can also be used to read the file without making any modifications, depending on the parameters passed to it.
fopen ( string $filename , string $mode [, bool $use_include_path = FALSE [, resource $context ]] ) : resource
The $filename
parameter is the file to be opened, for example, "/var/www/html"
. On a Windows server, you need to escape backslashes, like "c:\\myfolder\\myfile"
, by adding an extra \
in front of each one.
The $mode
parameter defines the mode in which the file is opened: r
(read) is for reading, and w
(write) is for writing. The mode specifies the type of access required for the stream. This does not mean the file is being read or written to yet—functions like fread()
or fwrite()
are needed for that.
Here are the available modes:
mode | Description |
---|---|
‘r’ | Opens for reading only, places the file pointer at the beginning. |
‘r+’ | Opens for reading and writing, places the file pointer at the beginning. |
‘w’ | Opens for writing only, truncates the file to zero length. Creates the file if it doesn’t exist. |
‘w+’ | Opens for reading and writing, truncates the file. Creates the file if it doesn’t exist. |
‘a’ | Opens for writing only, places the file pointer at the end. Creates the file if it doesn’t exist. |
‘a+’ | Opens for reading and writing, places the file pointer at the end. Creates the file if it doesn’t exist. |
‘x’ | Creates and opens for writing only. Fails if the file already exists. |
‘x+’ | Creates and opens for reading and writing. Fails if the file already exists. |
‘c’ | Opens for writing only, without truncation. Creates the file if it doesn’t exist. |
‘c+’ | Opens for reading and writing, without truncation. Creates the file if it doesn’t exist. |
‘e’ | Sets the close-on-exec flag on the file descriptor, available only on POSIX-compliant systems. |
Then there are 2 more optional parameters. I won’t address them at this moment, perhaps in a future review.
Reading a file with fread()
Well, let’s do a file reading example first.
We will need a text file to read, for example, 1.txt
which contains any text, in my case, the text is “hola a todos y gracias por preferirnos” (“hello everyone and thank you for choosing us”).
In the .php
file that will read 1.txt
:
<?php $file = fopen("/var/www/html/LF_php/files/1.txt","r"); echo fread($file,filesize("/var/www/html/LF_php/files/1.txt"));
With this, we should already obtain the text inside 1.txt. Remember that I am passing the path of the file from my PC.
Writing a file with fwrite()
Let’s create something much more elaborate. First, we’ll create a form that tells the user which file to read, then load its content into a textarea to be modified and saved.
Before starting, I must explain that I will not be uploading any file. I simply want to extract the file’s information, open it with fopen()
, display it in the textarea using fread()
, and, after making changes, write it back with fwrite()
.
fwrite ( resource $handle , string $string [, int $length ] ) : int
Have you thought about how to do it?
This is the way I did it: one index.php
file for the form, I used a read.php
file to read the file and display it in the textarea, and a write.php
file to write it back.
<form enctype="multipart/form-data" action="read.php" method="post"> <label>Plese select a file to read</label> <input type="file" name="f"> <input type="submit" value="confirm"> </form>read.php
<?php $filename = $_FILES['f']['name']; $filepath = __DIR__."/files/".$filename; //"/var/www/html/LF_php/files/1.txt" $file = fopen($filepath,"r"); echo "<form action=\"write.php\" method=\"post\">"; echo "<div><label>Change file</label></div>"; echo "<div><textarea name=\"content\" style=\"width:350px;\">"; echo fread($file,filesize($filepath)); echo "</textarea ></div>"; echo "<input type=\"hidden\" name=\"filepath\" value=$filepath>"; echo "<input type=\"submit\" value=\"confirm\">"; echo "</form>"; fclose($file); ?>write.php
<?php error_reporting(E_ALL); ini_set("display_errors", 1); $content = $_POST['content']; $file = fopen($_POST['filepath'],"w"); $writed = fwrite($file,$content); if(!$writed){ echo "<p>el archivo no se a podido escribir</p>"; }else{ echo "<p>el archivo se escribio con exito</p>"; } echo "<p><a href=\"index.php\">go back</a></p>";
In case you were working with Linux, here are the permissions you need:
~sudo chown -R www-data:www-data [tu ruta a la carpeta de trabajo] sudo chmod -R g+rw [tu ruta a la carpeta de trabajo]
Well, and how do we create the files? By using fopen
with mode ‘w+’, if the file doesn’t exist, it will try to create it. That’s an easy way to create a file.
When could I use fopen
?
At some point, we may create a system that generates files for our own project, so it’s not difficult to end up using fopen
to create files.
Other way – creating a file in PHP with file_put_contents
Another easy way to create a file with PHP is the file_put_contents
function. As the name suggests, this function is used to put data inside a file.
file_put_contents ( string $filename , mixed $data [, int $flags = 0 [, resource $context ]] ) : int
Although this function is more complex than fopen
, it has its advantages at certain points. For example, it allows adding data without overwriting the file in a much simpler way than fopen
, by using flags.
Flag | Description |
---|---|
FILE_USE_INCLUDE_PATH |
Searches for the $filename in the include directory. See more about include_path for details. |
FILE_APPEND |
If the file $filename already exists, appends the data to the file instead of overwriting it. |
LOCK_EX |
Acquires exclusive access to the file during the write operation. This means a call to flock() happens between fopen() and fwrite() . This is different from using fopen() with mode “x”. |
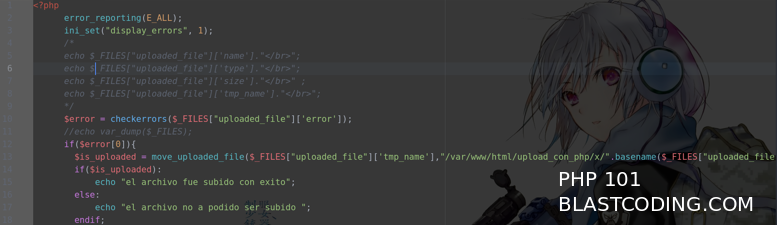
- Anterior: subiendo archivos con PHP
- Siguiente: Manejando fechas con PHP
You can make the code more readable just using <?php ?> or using the PHP heredoc, you not need to do the echo, echo, echo … thing